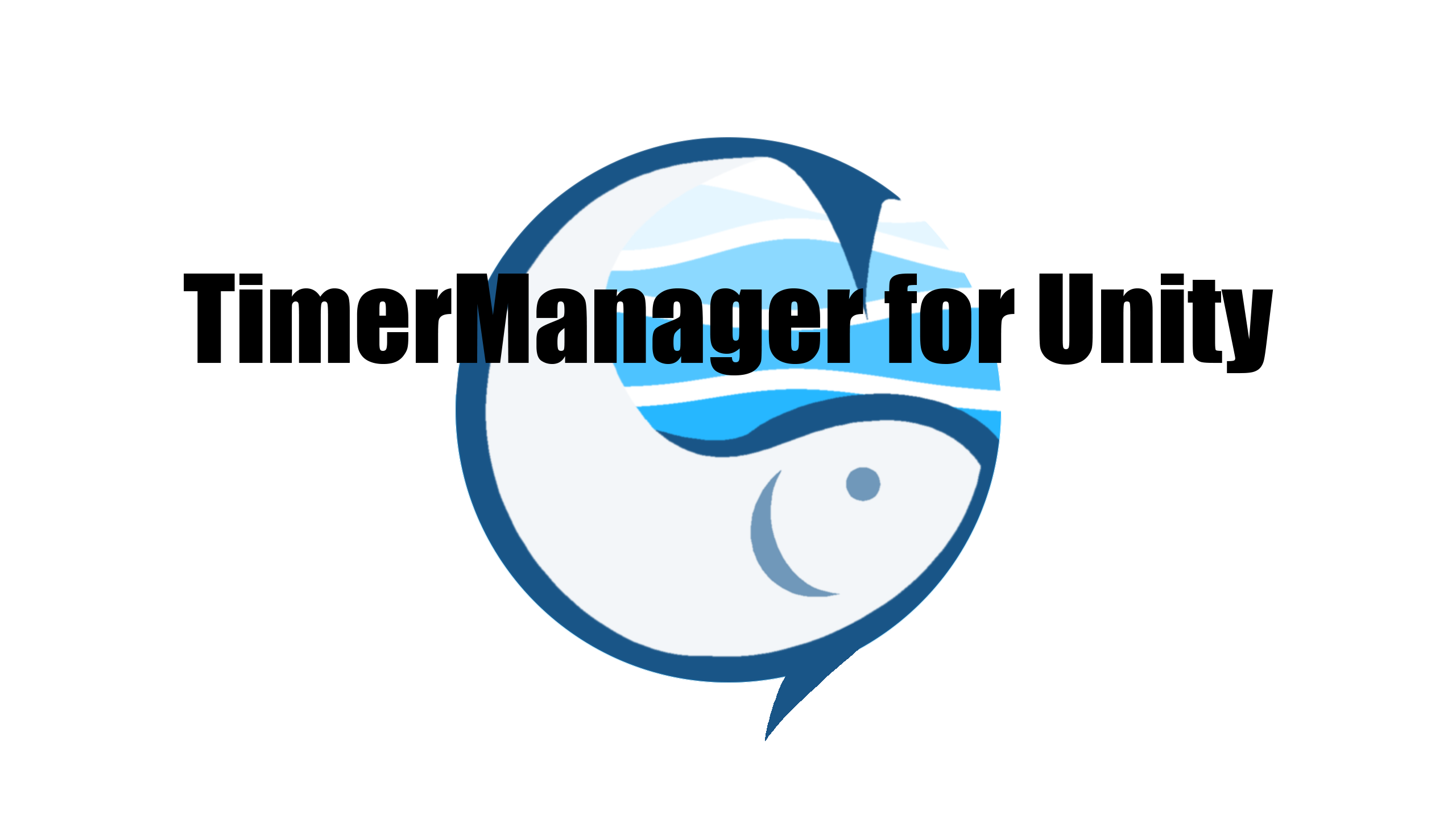
TimerManager for Unity is inspired by UE TimerManager, with performance optimizations and method expansions based on it.
No GC!!!
No GC!!!
No GC!!!
No garbage collection throughout the usage.
Entrance
Purchase
API-EN
API-CN
Quick Start
1. Set & Clear timer
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
| public class SampleSetTimerAndClearTimer : MonoBehaviour { public float Duration = 5f; private TimerHandle handle;
private void OnCheck(TimerHandle inHandle) { Debug.Log($"Delegate is excuted by Timer at time {Time.time}"); }
private void Update() { if (Input.GetKeyUp(KeyCode.S)) { TimerManager.GetInstance().SetTimer(ref handle, OnCheck, Duration); Debug.Log($"Set Timer at time {Time.time}"); } if (Input.GetKeyUp(KeyCode.C)) { TimerManager.GetInstance().ClearTimer(handle); Debug.Log("Clear Timer"); } } private void OnDestroy() { TimerManager.Instance?.ClearAllTimersForObject(this); } }
|
2. Pause & UnPause timer
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| public class SamplePauseAndUnPause : MonoBehaviour { public float Duration = 2f; private TimerHandle handle; private void OnCheck(TimerHandle inHandle) { Debug.Log($"Delegate is excuted by Timer at time {Time.time}"); } private void Start() { TimerManager.GetInstance().SetTimer(ref handle, OnCheck, Duration, inbLoop: true); } private void Update() { if (Input.GetKeyUp(KeyCode.P)) { TimerManager.GetInstance().PauseTimer(handle); Debug.Log($"Pause timer at time {Time.time}"); } if (Input.GetKeyUp(KeyCode.U)) { TimerManager.GetInstance().UnPauseTimer(handle); Debug.Log($"Unpause timer at time {Time.time}"); } } private void OnDestroy() { TimerManager.Instance?.ClearAllTimersForObject(this); } }
|
For other usage methods, please refer to the samples and API documentation provided with the plugin